Unityで配列を使いたい場合、
using UnityEngine;
public class Example : MonoBehaviour
{
public string[] Array;
}
のような感じで実装すると、Inspectorには次のように表示されます。
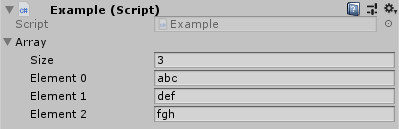
これだけでもInspectorから配列のサイズと値を設定することができて便利ですが、要素の並び替えがしたいと思って実装方法を調べてみました。
ReorderableList
ということで、ReorderableList
を使うとInspectorで並び替えができるようになるみたいです。
最低限の実装で並び替えだけしたい場合
とりあえず並び替えだけできれば良いという場合は、次のように実装できるみたいです。
using UnityEditor;
using UnityEditorInternal;
[CustomEditor(typeof(Example))]
public class ExampleEditor : Editor
{
ReorderableList _reorderableList;
void OnEnable()
{
var property = serializedObject.FindProperty("Array");
_reorderableList = new ReorderableList(serializedObject, property, true, true, false, false);
}
public override void OnInspectorGUI()
{
base.OnInspectorGUI();
serializedObject.Update();
_reorderableList.DoLayoutList();
serializedObject.ApplyModifiedProperties();
}
}
Inspectorの表示は次のような感じになります。
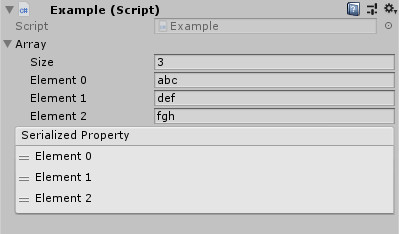
Serialized Propertyに表示されているElementをドラッグで移動できるようになります。(移動した場合は上のArrayの値も変更されます。)
値も表示したい
値も表示したいという場合は、次のような感じでdrawElementCallback
を実装します。
void OnEnable()
{
var property = serializedObject.FindProperty("Array");
_reorderableList = new ReorderableList(serializedObject, property, true, true, false, false);
_reorderableList.drawElementCallback = (rect, index, isActive, isFocused) =>
{
var element = property.GetArrayElementAtIndex(index);
EditorGUI.PropertyField(rect, element);
};
}
Inspectorの表示は次のような感じになります。
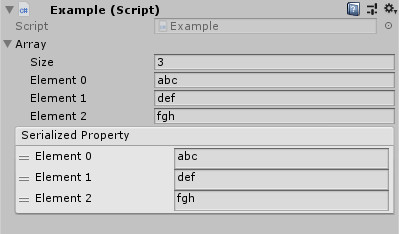
構造体を表示したい
構造体を表示したい場合は、Serializable
属性を設定した上で、次のような感じで修正します。
using UnityEngine;
public class Example : MonoBehaviour
{
[System.Serializable]
public struct Struct
{
public string text;
public int value;
}
public Struct[] Array;
}
using UnityEditor;
using UnityEditorInternal;
[CustomEditor(typeof(Example))]
public class ExampleEditor : Editor
{
ReorderableList _reorderableList;
void OnEnable()
{
var property = serializedObject.FindProperty("Array");
_reorderableList = new ReorderableList(serializedObject, property, true, true, false, false);
_reorderableList.elementHeightCallback = (index) =>
{
var element = property.GetArrayElementAtIndex(index);
return EditorGUI.GetPropertyHeight(element.FindPropertyRelative("text")) + EditorGUI.GetPropertyHeight(element.FindPropertyRelative("value"));
};
_reorderableList.drawElementCallback = (rect, index, isActive, isFocused) =>
{
var element = property.GetArrayElementAtIndex(index);
rect.height = EditorGUI.GetPropertyHeight(element.FindPropertyRelative("text"));
EditorGUI.PropertyField(rect, element.FindPropertyRelative("text"));
rect.y += rect.height;
rect.height = EditorGUI.GetPropertyHeight(element.FindPropertyRelative("value"));
EditorGUI.PropertyField(rect, element.FindPropertyRelative("value"));
};
}
public override void OnInspectorGUI()
{
base.OnInspectorGUI();
serializedObject.Update();
_reorderableList.DoLayoutList();
serializedObject.ApplyModifiedProperties();
}
}
Inspectorの表示は次のような感じになります。
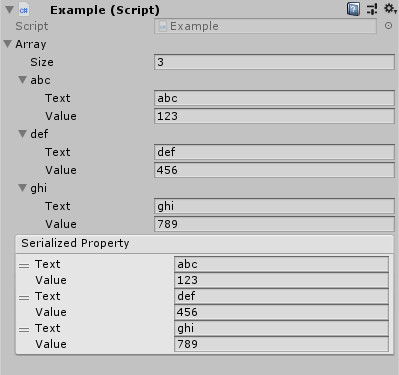
もう少し真面目に実装したい場合
同じ設定が二重に表示されないようにしたい場合はHideInInspector
属性を設定します。
[HideInInspector] public Struct[] Array;
ただ、このままではInspectorから配列のサイズが変更できなくなってしまうので、
_reorderableList = new ReorderableList(serializedObject, property);
とした上で、_reorderableList
にonAddCallback
とonRemoveCallback
を実装すると良いみたいです。
関連記事
UnityのCustomEditorでInspector表示を強制的に再描画する方法
UnityでCustomEditorを使っていて、内容が更新されているのにInspectorの表示が更新されないときはEditorUtility.SetDirty(target)を実行してみると良いそうです。
void OnInspectorGUI()
{
EditorUtility.SetDirty(target);
}
リンク
How do you force a cu...